Python Linear Search
Here you will get program for linear search in python.
Linear search is one of the simplest searching algorithm in which targeted item in sequentially matched with each item in a list. It is worst searching algorithm with worst case time complexity O (n).
Below is its implementation.
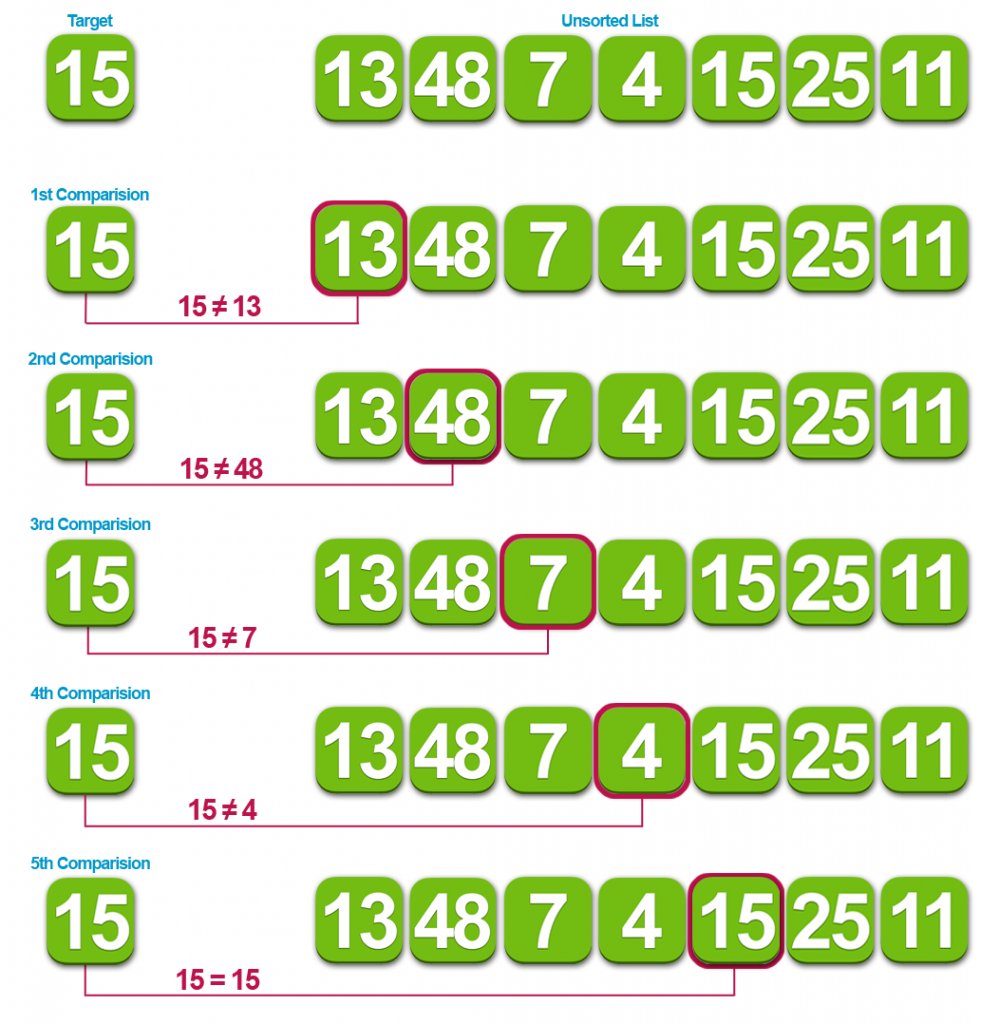
Program for Python Linear Search
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
|
items = [5, 7, 10, 12, 15]
print("list of items is", items)
x = int(input("enter item to search:"))
i = flag = 0
while i < len(items):
if items[i] == x:
flag = 1
break
i = i + 1
if flag == 1:
print("item found at position:", i + 1)
else:
print("item not found")
|
Output
list of items is [5, 7, 10, 12, 15]
enter item to search:12
item found at position: 4
enter item to search:12
item found at position: 4
Comment below if you have any queries regarding python linear search program.
Python Linear Search
linear search in python using recursion
estimating search time in python
binary search in python crazy programmer
linear search in c
python binary sort
python binary search recursion
linear search in java
linear search in dictionary python
Tags:
python